Canny Edge Detector
The Canny edge detector is an edge detection algorithm that uses a multi-stage algorithm to detect a wide range of edges in images. It was developed by John F. Canny in 1986. The algorithm is based on grayscale pictures. Therefore, the prerequisite is to convert the image to grayscale before performing the Canny edge detection.
The Canny edge detection algorithm is composed of 5 steps:
- Noise reduction;
- Gradient calculation;
- Non-maximum suppression;
- Double threshold;
- Edge Tracking by Hysteresis.
Since the Canny edge detector is based on gradient, the result is highly sensitive to image noise. So it is also a good practice to apply a blurring to the gray scaled image before the Canny edge detection.
In this example code below, we preprocess the image with gray scale and Gaussian blurring. Then we feed the preprocessed image to the canny edge detector as shown below. After that, we could extract the contours using the findContours and drawContours methods to reproduce the contours with desired background and color.
import cv2 as cv
import numpy as np
img = cv.imread('./images/pokemon.jpg')
cv.imshow('Original', img)
gray = cv.cvtColor(img, cv.COLOR_BGR2GRAY)
cv.imshow('Gray', gray)
blur = cv.GaussianBlur(gray, (5,5), cv.BORDER_DEFAULT)
cv.imshow('Blur', blur)
canny = cv.Canny(blur , 120, 155)
cv.imshow('Canny Edges', canny)
contours, hierarchies = cv.findContours(canny, cv.RETR_LIST, cv.CHAIN_APPROX_SIMPLE)
print(f'{len(contours)} contour(s) created.')
blank = np.zeros(img.shape, dtype='uint8')
cv.drawContours(blank, contours, -1, (0,0,255), 1)
cv.imshow('Contours Drawn', blank)
cv.waitKey(0)
Original image before Canny Edge detection:
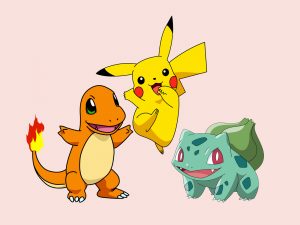
Output image after Canny Edge detection:

References:
- Canny Edge Detection Step by Step in Python — Computer Vision
- OpenCV Course – Full Tutorial with Python