Text Recognition Using Google Cloud Vision API
You can readily use Google Cloud API to extract text from an image using Google Cloud Vision
The steps are as follows:
- Step 1: Create a project in Google Cloud console
- Step 2: Enable Google Cloud Vision AI
- Step 3: Create a service account
- Step 4: Create a service key and download the key
After that, you can open a new Google Colab file and install the Google Cloud API package
!pip install google-cloud-vision
Upload and image with text
path = r'/content/starter.jpg'
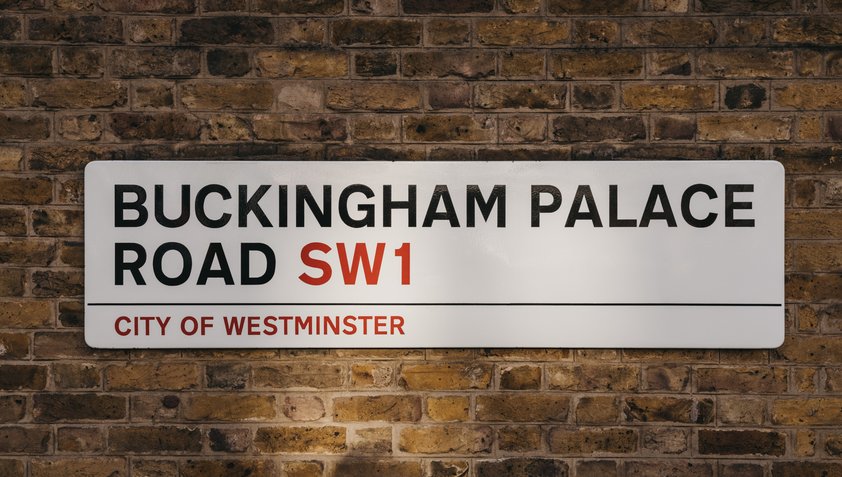
Run the following code using Google Cloud Vision API
import os, io
from google.cloud import vision
#from google.cloud.vision import types
from google.cloud.vision_v1 import types
os.environ['GOOGLE_APPLICATION_CREDENTIALS'] = r'service-account.json'
def starter(path):
client = vision.ImageAnnotatorClient()
if path.startswith('http') or path.startswith('gs:'):
image = types.Image()
image.source.image_uri = path
else:
with io.open(path, 'rb') as image_file:
content = image_file.read()
image = types.Image(content=content)
result = client.text_detection(image=image).text_annotations
return result
def get_text(texts):
Output:
BUCKINGHAM PALACE
ROAD SW1
CITY OF WESTMINSTER
BUCKINGHAM
PALACE
ROAD
SW1
CITY
OF
WESTMINSTER
References:
Relevant Courses
May 30, 2021